class: middle, center # The Internet 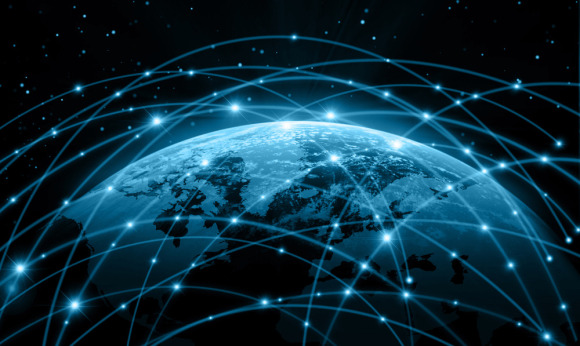 [http://pjb3.me/bewd-internet](http://pjb3.me/bewd-internet) .footnote[ created with [remark](http://github.com/gnab/remark) ] --- class: middle, center # How does it work?  --- # IP Addresses All computers on the Internet have an Internet Protocol (IP) Address They look like this: 50.57.195.189 IP addresses are too hard to memorize and they change a lot anyway --- # DNS The Domain Name System (DNS) allows us to use names instead of numbers The process of looking up the actual IP address for a domain name is called __resolution__ .terminal $ dig betamore.com +short 50.57.195.189 `dig` is a command line tool that allows you to see what IP address a domain name resolves to --- # URLs Uniform Resource Location (URL) is a reference to a page on the internet .terminal http://betamore.com/academy/back-end-web-development.html `http` is the protocol to use to retrieve the document `betamore.com` is the domain of the server that is hosting this document `/academy/back-end-web-development` is the path to the document Run a simple web server with this command: .terminal ruby -run -ehttpd /var/www/betamore -p8000 Directory structure .terminal / └── var └── www └── betamore └── academy └── back-end-web-development.html --- # HTTP __HyperText Transfer Protocol__ (HTTP) is the underlying protocol of the Internet. You can issue an HTTP request from the command line like this: .terminal $ curl -svo /dev/null http://betamore.com/academy/back-end-web-development/ `curl` is a tool that allow you to send HTTP requests and receive the response It is like a web browser that doesn't render the HTML document There are headers in the request and the response --- # HTTP POST Install Firefox & HTTPFox (Tools > Add-ons). HTTPFox is an add on that allows you to see the HTTP traffic sent back and forth. Put the following code into post.html ```html
POST example
Credit Card
``` .terminal $ open -a Firefox.app post.html Open HTTPFox in a new window (Tools > Web Developer > HttpFox > Open In Own Window), start HTTPFox (green play button), and submit the form --- # POST Data 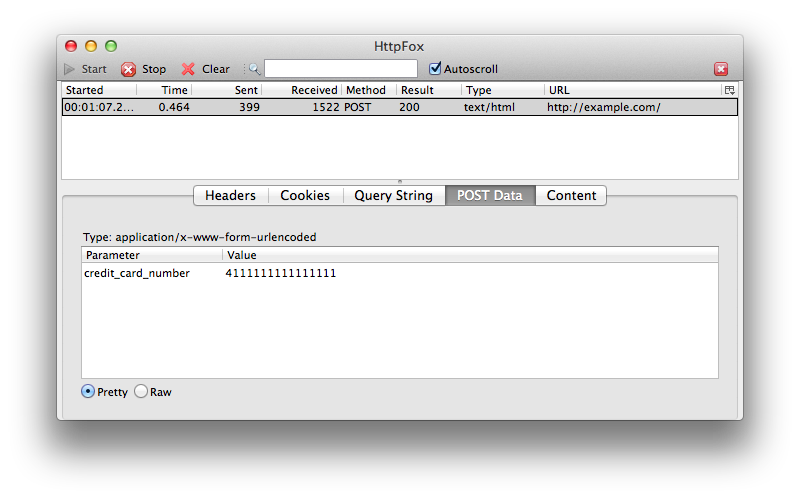 --- # Raw HTTP POST Doing the same thing in curl would look like this: .terminal POST / HTTP/1.1 User-Agent: curl/7.24.0 Host: example.com Accept: */* Content-Length: 35 Content-Type: application/x-www-form-urlencoded credit_card_number=4111111111111111 __Problem__: Anyone on the network can intercept these requests! --- # Security HTTPS, also known as Secure HTTP, uses a Secure Socket Layer (SSL) to encrypt HTTP requests/responses .center[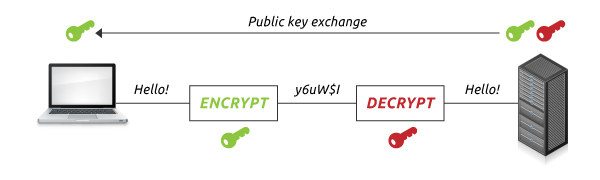] The browser will use SSL for URLs with the `https` protocol We can build a demonstration of what encryption looks like using Ruby --- # Public/Private Key Encryption Generate your own public/private key pair .terminal $ openssl genrsa -out mykey.pem 512 Generating RSA private key, 512 bit long modulus ...++++++++++++ .....++++++++++++ e is 65537 (0x10001) Extract the public key from the key pair .terminal $ openssl rsa -in mykey.pem -pubout > mykey.pub writing RSA key --- # Public / Private Key Encryption The private key .terminal $ cat mykey.pem -----BEGIN RSA PRIVATE KEY----- MIIBOQIBAAJBAPhtyQ8MkUroCBU5skqHj9gofp9CYVwb/9p/tadiRym1oE4LNRh6 tBh4bFggBUpycwostGT4qly5cPXuUc30iy8CAwEAAQJAe5Joe2Ld9quaMaykYewy gewKp/9l3GJVDwONgTgie7zQoJow8U6FgpicS8UQzwyv76rgPs8d9mqv70UUE8on mQIhAPyuO7epHS2I9sVAqhi2MOLlYjg2qgscoJ9ZkMT7fpJ1AiEA+7FA4g85qZOA sAiBdKWtksePxNTbzBo/jAqZELTaapMCIGJU66hth41Q37ejko5LWHr0CWUW4NWy Lk/U6yG9PWSBAiAkOSZ1YmuSFEB5FUBMYWvRrBbs5RWdEkoDBaNx3BVzMQIgLc/x V2gHi0Rexg2rUlrO9lprEIUwLKNAHHnubImGISY= -----END RSA PRIVATE KEY----- The public key .terminal $ cat mykey.pub -----BEGIN PUBLIC KEY----- MFwwDQYJKoZIhvcNAQEBBQADSwAwSAJBAPhtyQ8MkUroCBU5skqHj9gofp9CYVwb /9p/tadiRym1oE4LNRh6tBh4bFggBUpycwostGT4qly5cPXuUc30iy8CAwEAAQ== -----END PUBLIC KEY----- --- # Public / Private Key Encryption Put this code into `pub_priv_key.rb`: ```ruby require 'openssl' require 'base64' data = 'credit_card_number=4111111111111111' puts "Encrypting data: (this is what would be entered into the browser)" puts data puts # Everyone on the Internet gets access to this public key public_key = OpenSSL::PKey::RSA.new(File.read("mykey.pub")) encrypted_data = Base64.encode64(public_key.public_encrypt(data)) puts "Encrypted data: (this is what would be sent to the server)" puts encrypted_data puts # This file is kept a secret on the server of example.com private_key = OpenSSL::PKey::RSA.new(File.read("mykey.pem")) decrypted_data = private_key.private_decrypt(Base64.decode64(encrypted_data)) puts "Decrypted data: (this is what the server gets after decryption)" puts decrypted_data puts ``` --- # Public / Private Key Encryption .terminal $ ruby pub_priv_key.rb Encrypting data: (this is what would be entered into the browser) credit_card_number=4111111111111111 Encrypted data: (this is what would be sent to the server) qaaOj7kg7kxQ2OgYGzqFtZIjx4Rdve1q7lJkbz6wVKRLzKjXfP+iQQ4kFmjz N55fQ7WHBjXK38g0to9yOsxJyQ== Decrypted data: (this is what the server gets after decryption) credit_card_number=4111111111111111 --- # Making our form secure Use **https** as the protocol in the action instead of **http**: ```html
POST example
Credit Card
```